About
Smoke out is a first person shooter which focusses on defeating AI enemies which take on the roles of a drugs cartel. As a police officer you must defeat all the cartel members and secure the objectives to win.
Project Info
Group Size: 3
Project Length: 4 Weeks
Project Date: February 2024
Engine & Tools: Unity, Visual Studio Code & Trello
Finite State Machines
In this project, I took charge of designing and implementing the enemy AI and behavior, a task that allowed me to get a better understanding of game AI. One of the key components I developed was a finite state machine (FSM) to manage the behavior of cartel members within the game.
Rather than settling for conventional enemy AI that simply advances towards the player or wanders aimlessly, I sought to create a more dynamic and engaging experience. My solution involved crafting a system where AI characters prioritize seeking cover before strategically peeking around corners to take shots at the player.
Here you can see the enemies getting a cover position and entering the “taking cover” state.
Partial AI Movement Code
private void LookForCoverState(AState pState, float pDeltaTime)
{
CheckForDisengage();
if (!_coverIsFound)
{
_newCover = AISeekCover.Instance.GetCover(Target.transform.position);
_coverIsFound = true;
}
if (_newCover == null)
{
_stateMachine.Goto(EStates.Wander);
return;
}
// Set the location for the AI and move towards it
_animator.SetBool("IsWalking", true);
Vector3 locationToGo = _newCover.transform.position;
_agent.SetDestination(locationToGo);
ApplyRotation();
float distance = Vector3.Distance(transform.position, locationToGo);
// If target arrived at cover
if (distance <= 2)
{
_animator.SetBool("IsWalking", false);
_stateMachine.Goto(EStates.LookForEnemy);
return;
}
}
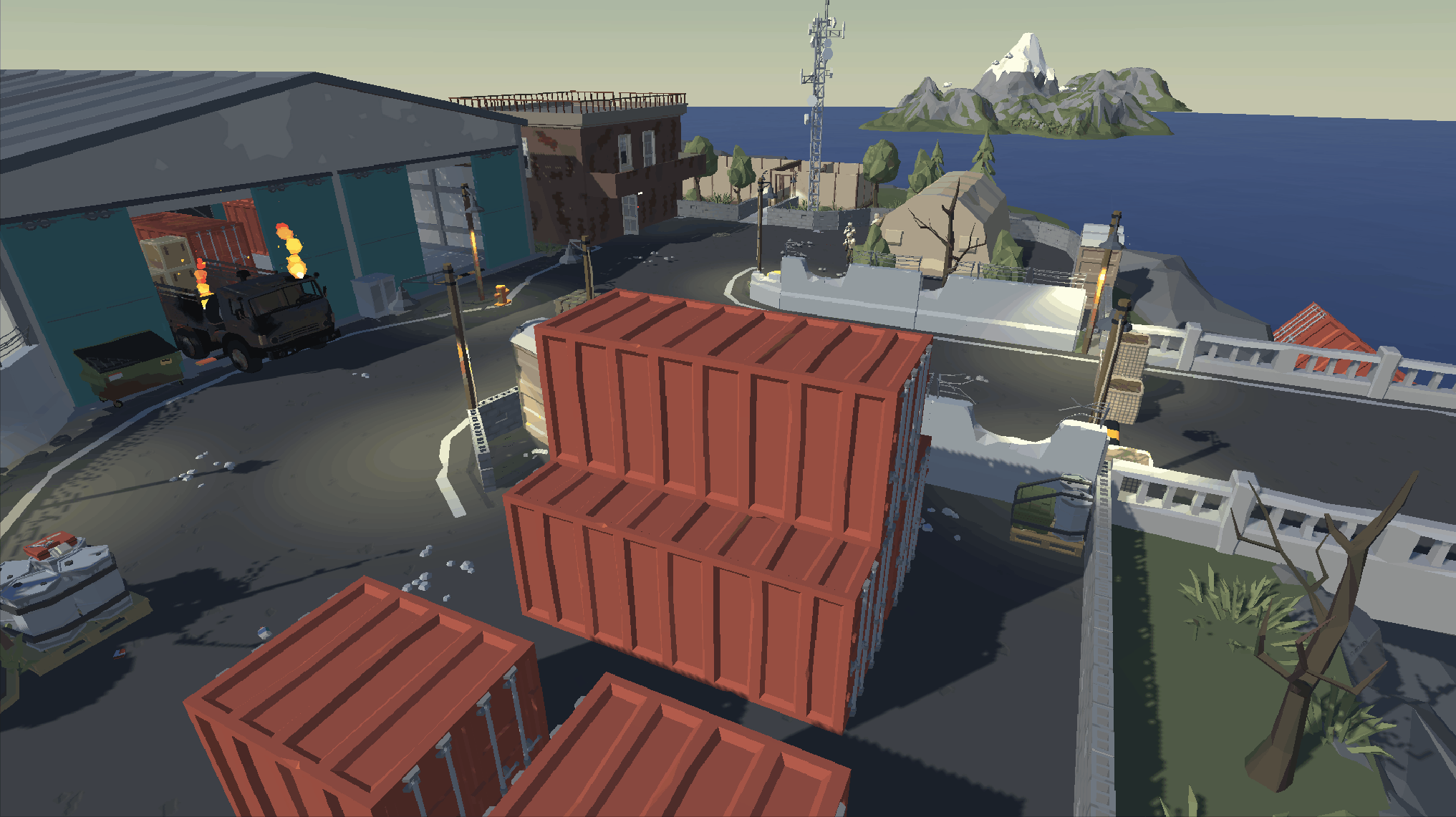
Here you can see the enemies when they reach a cover position. They decide to shoot at the target, and take cover after firing for a while.
Partial AI Shooting Code
private void ShootState(AState pState, float pDeltaTime)
{
CheckForDisengage();
RotateTowardsTarget();
_animator.SetBool("TakingCover", false);
_animator.SetBool("AbleToShoot", true);
_gun.CanShoot = true;
if (_targetToShoot == null)
{
return;
}
_gun.SetTarget(_targetToShoot.transform);
// Checks if this state is active for _MinTimer amount of time
if (pState.Lifetime < _MinTimer)
{
return;
}
else if (pState.Lifetime > _MinTimer)
{
_stateMachine.Goto(EStates.TakeCover);
}
}
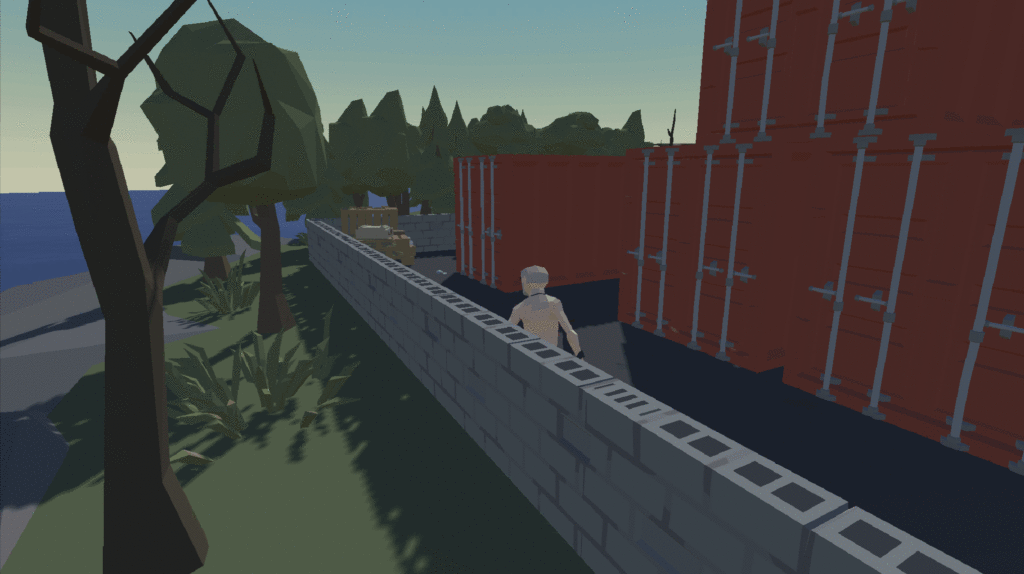
By implementing this FSM-based AI, I worked on my ability to make enemy AI and worked with this type of system for the first time. This project served as a stepping stone for my knowledge about AI and i have a greater knowledge about how Finite State Machines work.