About
Roman Revenant is a top down singleplayer game where you play as a Roman warrior defending the city of Rome from invading monsters. As you progress, you unlock a variety of weapons, each with unique attributes and combat styles, allowing you to customize your strategy. Additionally, you gain special abilities tailored to each weapon.
Project Info
Group Size: 7
Project Length: 8 Weeks
Project Date: April 2024
Engine & Tools: Unity, Visual Studio Code & Trello
Summary
Player Combat
Player Abilities
Player Movement
Enemy Wave Spawning
Player Combat & Ability System In this project, I was responsible for designing all aspects related to the player, including movement and combat. One of the key components of the player was the combat system, which I divided into two separate parts: Player Combat and Player Abilities.
For the combat, I chose a simple yet effective approach. I created a system where the player has linked box colliders used as hitboxes. Different weapons have different sizes and corresponding hitboxes. The sizes are based on the appearance of the weapon; for example, the spear has a long but slim hitbox, while the battleaxe has a short but wide hitbox.
In this system, I used references to hitboxes for light and heavy attacks, each with its own hitbox and animation. When an attack button is pressed, the hitboxes for that attack are enabled, allowing enemies to take damage.
Partial Player Combat Code
public class PlayerCombat : MonoBehaviour
{
[Header("References")]
private PlayerController playerController;
private WeaponCollision weaponCollision;
private Animator animator;
[Header("Animations")]
[SerializeField] private float animationDuration;
[Header("Input")]
[SerializeField] private KeyCode lightAttack;
[SerializeField] private KeyCode heavyAttack;
private void Start()
{
animator = GetComponent<Animator>();
playerController = GetComponent<PlayerController>();
weaponCollision = GetComponentInChildren<WeaponCollision>();
}
private void Update()
{
LightAttack();
HeavyAttack();
}
private void LightAttack()
{
if (Input.GetKeyDown(lightAttack))
{
AnimatorClipInfo[] clipInfo = animator.GetCurrentAnimatorClipInfo(0);
if (clipInfo.Length > 0)
{
StartAttack(0, "LightAttack");
}
}
}
private void HeavyAttack()
{
if (Input.GetKeyDown(heavyAttack))
{
AnimatorClipInfo[] clipInfo = animator.GetCurrentAnimatorClipInfo(0);
if (clipInfo.Length > 0)
{
StartAttack(20, "HeavyAttack");
}
}
}
private void StartAttack(float staminaCostForAttack, string attackType)
{
animator.SetTrigger(attackType);
playerController.DrainStaminaOnAttack(staminaCostForAttack);
if (attackType == "LightAttack")
{
weaponCollision.EnableLightHitbox();
}
else if (attackType == "HeavyAttack")
{
weaponCollision.EnableHeavyHitbox();
}
StartCoroutine(WaitForAttack());
}
private IEnumerator WaitForAttack()
{
playerController.CanMove = false;
yield return new WaitForSeconds(animationDuration);
ResetAttack();
}
private void ResetAttack()
{
animator.ResetTrigger("HeavyAttack");
animator.ResetTrigger("LightAttack");
weaponCollision.DisableHitboxes();
playerController.CanMove = true;
}
}
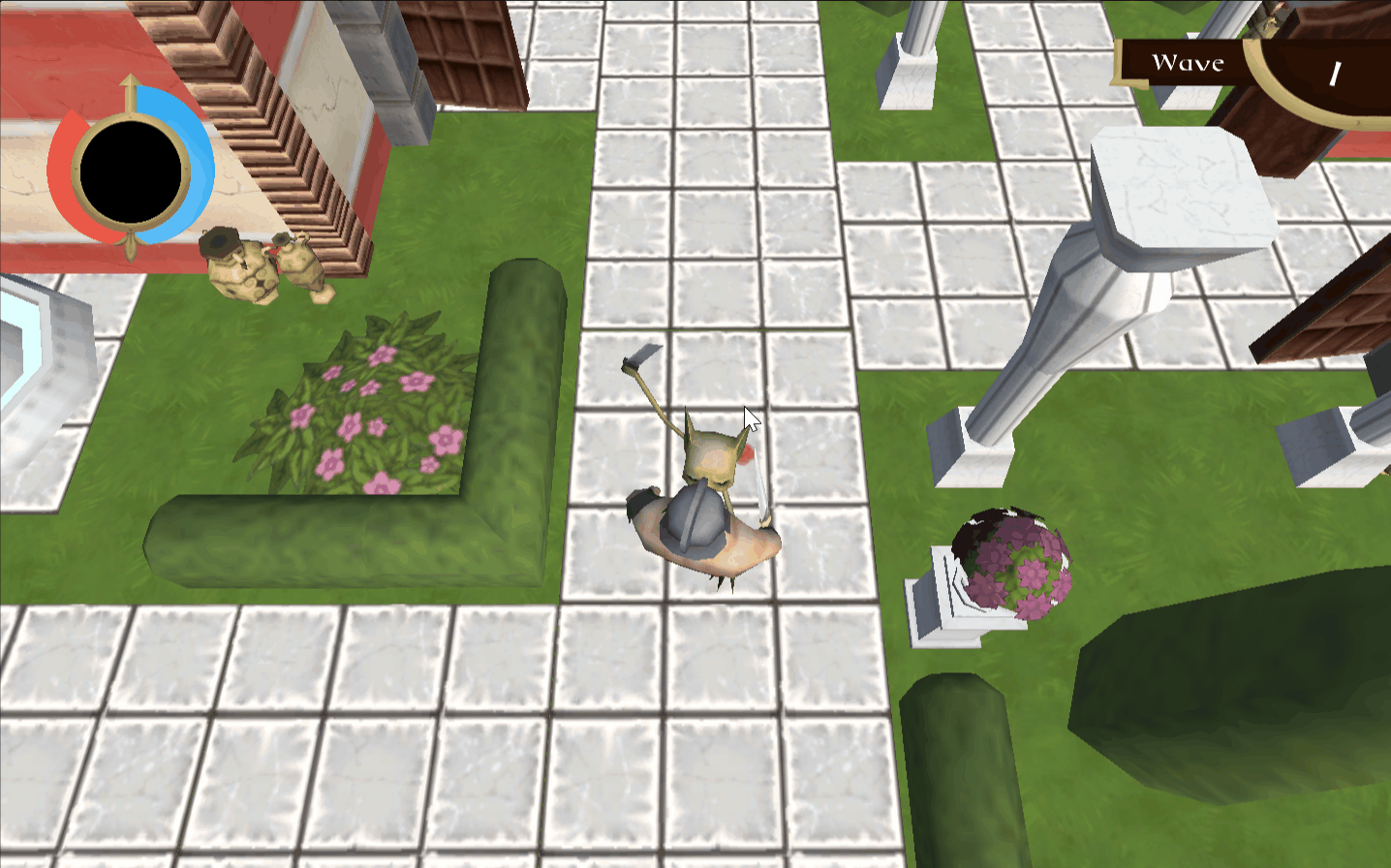
For abilities, I decided to base them of the players current weapon. The PlayerUseAbilities
class contains a dictionary of abilities linked to weapon types and tracks cooldowns to manage ability usage. The InitializeAbilityDictionary method sets up abilities like healing for the sword, special attacks for the axe, defensive moves for the shield, and ranged attacks for the spear. The UseAbility
method checks for cooldowns and stamina before activating an ability. The script includes an abstract Ability
class and specific implementations such as SwordAbility, AxeAbility, ShieldAbility, and SpearAbility, each with unique functions and animations.
Partial Player Ability Code
public class PlayerUseAbilities : MonoBehaviour
{
private PlayerController playerController;
private ActivateWeapon activateWeapon;
private Dictionary<string, Ability> abilityDictionary;
private Dictionary<string, float> abilityCooldowns;
private string currentWeapon;
[SerializeField] private GameObject spearThrowPrefab;
[SerializeField] private Transform spearThrowOrigin;
private void Start()
{
InitializeAbilityDictionary();
playerController = GetComponent<PlayerController>();
activateWeapon = GetComponent<ActivateWeapon>();
abilityCooldowns = new Dictionary<string, float>();
currentWeapon = activateWeapon.HoldingWeapon.ToString();
}
private void InitializeAbilityDictionary()
{
abilityDictionary = new Dictionary<string, Ability>
{
{ "Sword", new SwordAbility(50, 15f, 30f) }, // HealAmount Cooldown Cost
{ "Axe", new AxeAbility(12f, 30f) }, // Cooldown Cost
{ "Shield", new ShieldAbility(16f, 30f) }, // Cooldown Cost
{ "Spear", new SpearAbility(7f, 15f, spearThrowPrefab, spearThrowOrigin) } // Cooldown Cost WeaponPrefab Origin
};
}
private void Update()
{
if (Input.GetKeyDown(KeyCode.Q))
{
UseAbility(currentWeapon);
}
}
private void UseAbility(string weaponType)
{
if (abilityDictionary.ContainsKey(weaponType))
{
Ability ability = abilityDictionary[weaponType];
float lastUsedTime;
if (abilityCooldowns.TryGetValue(weaponType, out lastUsedTime))
{
if (Time.time < lastUsedTime + ability.Cooldown)
{
Debug.Log("Ability on Cooldown");
return;
}
}
if (playerController.Stamina < ability.StaminaCost)
{
Debug.Log("Not enough stamina to use Ability");
return;
}
ability.Use(gameObject);
abilityCooldowns[weaponType] = Time.time;
playerController.DrainStamina(ability.StaminaCost);
if (ability is AxeAbility axeAbility)
{
StartCoroutine(axeAbility.WaitForAbilityCoroutine(gameObject));
}
else if (ability is ShieldAbility shieldAbility)
{
StartCoroutine(shieldAbility.WaitForAbilityCoroutine(gameObject));
StartCoroutine(shieldAbility.RollCoroutine());
}
else if (ability is SpearAbility spearAbility)
{
StartCoroutine(spearAbility.WaitForSpearAbility(gameObject));
}
}
else
{
Debug.LogWarning("No Ability found for weapon type: " + weaponType);
}
}
private abstract class Ability
{
public float Cooldown { get; private set; }
public float StaminaCost { get; private set; }
protected Ability(float cooldown, float staminaCost)
{
Cooldown = cooldown;
StaminaCost = staminaCost;
}
public abstract void Use(GameObject user);
}
private class SwordAbility : Ability
{
private int healAmount;
public SwordAbility(int healAmount, float cooldown, float staminaCost)
: base(cooldown, staminaCost)
{
this.healAmount = healAmount;
}
public override void Use(GameObject user)
{
PlayerHealth playerHealth = user.GetComponent<PlayerHealth>();
if (playerHealth != null)
{
playerHealth.Heal(healAmount);
}
}
}
private class AxeAbility : Ability
{
public AxeAbility(float cooldown, float staminaCost)
: base(cooldown, staminaCost) { }
private WeaponCollision weaponCollision;
private Animator animator;
public override void Use(GameObject user)
{
weaponCollision = user.GetComponentInChildren<WeaponCollision>();
animator = user.GetComponent<Animator>();
animator.SetTrigger("AbilityAttack");
weaponCollision.EnableAbilityHitbox();
}
public IEnumerator WaitForAbilityCoroutine(GameObject user)
{
yield return new WaitForSeconds(1.6f);
weaponCollision.DisableHitboxes();
}
}
private class ShieldAbility : Ability
{
private CharacterController characterController;
private WeaponCollision weaponCollision;
private Animator animator;
private float rollDistance = 8;
private float rollSpeed = 20;
private Vector3 movement;
public ShieldAbility(float cooldown, float staminaCost)
: base(cooldown, staminaCost) { }
public override void Use(GameObject user)
{
weaponCollision = user.GetComponentInChildren<WeaponCollision>();
characterController = user.GetComponent<CharacterController>();
animator = user.GetComponent<Animator>();
animator.SetTrigger("AbilityAttack");
weaponCollision.EnableAbilityHitbox();
float horizontal = Input.GetAxis("Horizontal");
float vertical = Input.GetAxis("Vertical");
movement.Set(horizontal, 0f, vertical);
}
public IEnumerator RollCoroutine()
{
Vector3 rollDirection = movement.normalized * rollDistance;
float rollTime = rollDistance / rollSpeed;
float elapsedTime = 0f;
while (elapsedTime < rollTime)
{
characterController.Move(rollDirection * Time.deltaTime / rollTime);
elapsedTime += Time.deltaTime;
yield return null;
}
characterController.Move(rollDirection * (rollTime - elapsedTime) / rollTime);
}
public IEnumerator WaitForAbilityCoroutine(GameObject user)
{
yield return new WaitForSeconds(0.8f);
weaponCollision.DisableHitboxes();
}
}
private class SpearAbility : Ability
{
private GameObject spearThrowPrefab;
private Transform spearThrowOrigin;
private Animator animator;
public SpearAbility(float cooldown, float staminaCost, GameObject spearThrowPrefab, Transform spearThrowOrigin)
: base(cooldown, staminaCost)
{
this.spearThrowPrefab = spearThrowPrefab;
this.spearThrowOrigin = spearThrowOrigin;
}
public override void Use(GameObject user)
{
if (spearThrowPrefab == null || spearThrowOrigin == null)
{
Debug.LogError("Spear throw prefab or origin is not set");
return;
}
animator = user.GetComponent<Animator>();
animator.SetTrigger("AbilityAttack");
}
public IEnumerator WaitForSpearAbility(GameObject user)
{
yield return new WaitForSeconds(0.45f);
Quaternion rotation = Quaternion.Euler(0, user.transform.rotation.eulerAngles.y, 0);
Instantiate(spearThrowPrefab, spearThrowOrigin.transform.position, rotation);
Quaternion rotation1 = Quaternion.Euler(0, user.transform.rotation.eulerAngles.y + 15, 0);
Instantiate(spearThrowPrefab, spearThrowOrigin.transform.position, rotation1);
Quaternion rotation2 = Quaternion.Euler(0, user.transform.rotation.eulerAngles.y - 15, 0);
Instantiate(spearThrowPrefab, spearThrowOrigin.transform.position, rotation2);
}
}
}
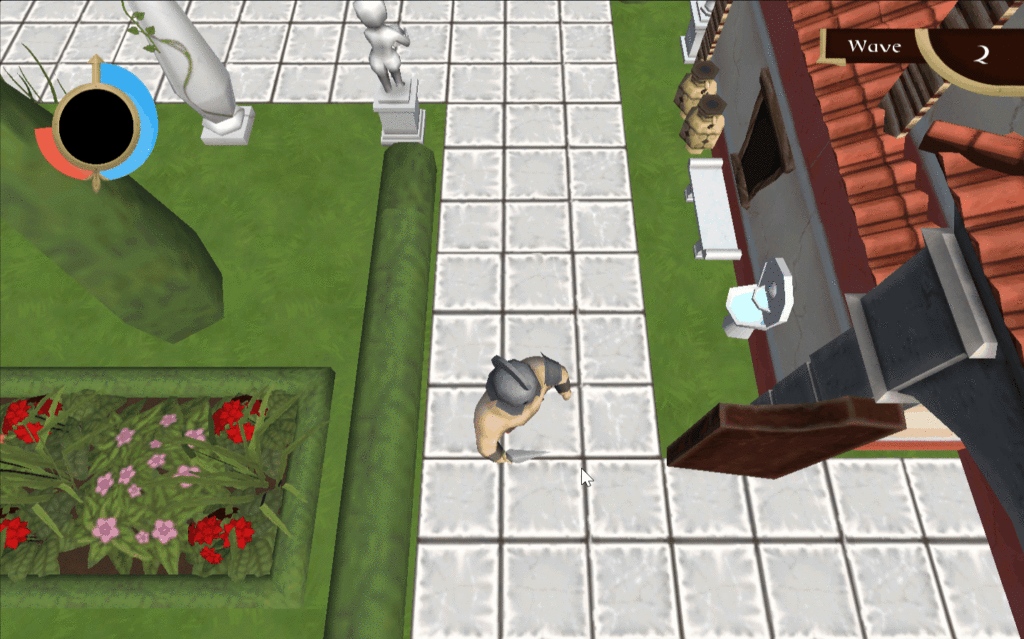
Player Movement
For the player movement i decided on making a simple but effective charachter controller using the inbuild Character Controller component. I made a simple script around it which i could change later into a more complex controller featuring dashes and stamina.
We wanted to leave physics out of this project so i move the player using the inbuild Move method that comes with the Character Controller component.
Partial Character Controller Code
private void MoveCharacter()
{
float horizontal = Input.GetAxis("Horizontal");
float vertical = Input.GetAxis("Vertical");
movement.Set(horizontal, 0f, vertical);
if (movement.magnitude > 1f)
{
movement.Normalize();
}
animator.SetBool("IsMoving", true);
characterController.Move(movement * moveSpeed * Time.deltaTime);
}
public void DrainStamina(float staminaAmount)
{
if (Stamina <= staminaAmount)
{
Debug.Log("Not enough stamina to perform this action");
return;
}
else
{
Stamina -= staminaAmount;
float currentStamina = Stamina / 100;
staminaBar.fillAmount = currentStamina;
lastStaminaUsedTime = Time.time;
}
}
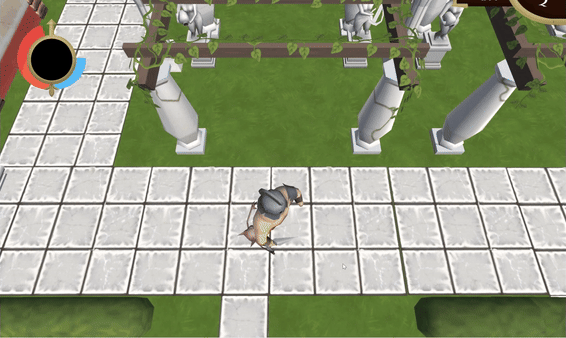
Wave System
One of my other developers was running late on making the enemies so i stepped in making a system to spawn enemies based on a weight system per wave. First i had calculate how much weight each wave had, how much weight every enemy has and how to increase the weight per wave.
My first step was making sure there could only be one Instance of the wave manager using an Singleton Reference. Then i decided to use a exponential graph to calculate the weight for each wave. Using that i made sure that when i spawned them i did not use the standard Random method that is inbuild in unity but rather make something that ensures there is a higher chance of getting higher weight enemies in higher waves.
Partial Wave System Code
private int CalculateWaveWeight(int waveNumber)
{
return currentWaveWeight = Mathf.RoundToInt(10 * Mathf.Pow(1.2f, waveNumber - 1));
}
private IEnumerator SpawnNextWave()
{
isSpawningWave = true;
CalculateWaveWeight(CurrentWave);
enemyManager.buffedEnemyCount = 0;
int totalWeightLeft = currentWaveWeight;
while (totalWeightLeft > 0)
{
EnemyTypes.Data selectedEnemy = SelectRandomEnemyType();
while (selectedEnemy.EnemyWeight > totalWeightLeft)
{
List<EnemyTypes.Data> suitableEnemies = new List<EnemyTypes.Data>();
foreach (EnemyTypes.Data enemyData in enemyTypesData.Enemies)
{
if (enemyData.EnemyWeight <= totalWeightLeft)
{
suitableEnemies.Add(enemyData);
}
}
if (suitableEnemies.Count > 0)
{
selectedEnemy = suitableEnemies[Random.Range(0, suitableEnemies.Count)];
}
else
{
break;
}
}
if (selectedEnemy.EnemyWeight > totalWeightLeft)
{
break;
}
Transform spawnPoint = spawnLocations[Random.Range(0, spawnLocations.Count)];
Instantiate(selectedEnemy.EnemyPrefab, spawnPoint.position, Quaternion.identity);
yield return new WaitForEndOfFrame();
uglyfix.Instance.UpdateNumber(CurrentWave);
totalWeightLeft -= selectedEnemy.EnemyWeight;
}
yield return new WaitUntil(() => CheckEnemyAmount());
CurrentWave++;
isSpawningWave = false;
}
private EnemyTypes.Data SelectRandomEnemyType()
{
int totalWeight = 0;
foreach (EnemyTypes.Data enemyData in enemyTypesData.Enemies)
{
totalWeight += enemyData.EnemyWeight;
}
int randomValue = Random.Range(0, totalWeight);
int cumulativeWeight = 0;
foreach (EnemyTypes.Data enemyData in enemyTypesData.Enemies)
{
cumulativeWeight += enemyData.EnemyWeight;
if (randomValue < cumulativeWeight)
{
return enemyData;
}
}
return enemyTypesData.Enemies[0];
}